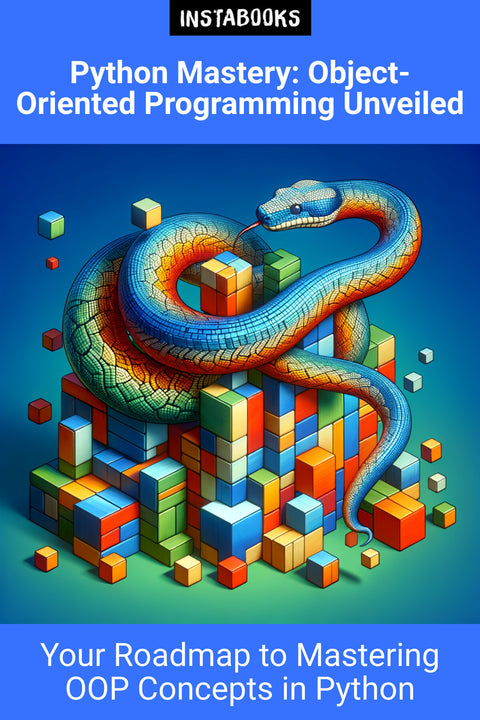
Python Mastery: Object-Oriented Programming Unveiled
Your Roadmap to Mastering OOP Concepts in Python
AI Textbook - 100+ pages



Embark on a journey through the intricacies of Object-Oriented Programming (OOP) in the versatile world of Python with 'Python Mastery: Object-Oriented Programming Unveiled'. Perfect for developers and enthusiasts at all levels, from beginners to experts, this comprehensive guide delves deep into OOP concepts, coupled with practical Python applications.
Discover the building blocks of OOP, such as classes, objects, and methods, and how they come to life in Python's ecosystem. Each concept is illuminated with clear explanations for newcomers while progressively revealing advanced theories for seasoned programmers. The book is designed to equip you with the foundational skills necessary to architect robust Python applications and inspire you to explore the elegance of OOP design patterns.
The author, a seasoned Python developer with years of experience, shares practical insights and tips to enhance your coding practice. Whether your goal is to refine your programming techniques, develop scalable applications, or innovate with Python's OOP capabilities, this book serves as a key educational resource.
Enjoy a well-structured exploration across 12 chapters, each focusing on different OOP aspects, ensuring a rich, layered learning experience. Dive into interactive examples, case studies, and projects that reinforce your understanding, moving you swiftly from theoretical knowledge to practical mastery.
'Python Mastery: Object-Oriented Programming Unveiled' isn't just a book; it's a pathway to elevating your programming skills through the lens of OOP in Python. Get ready to transform your ideas into reality with the power of object-oriented design!
Table of Contents
1. Foundations of OOP in Python- Understanding Objects and Classes
- The Pillars of OOP: Encapsulation
- Diving into Methods and Messages
2. The Anatomy of Python Classes
- Defining and Instantiating Classes
- Exploring Class and Instance Variables
- Class Methods, Static Methods, and Properties
3. Inheritance and Polymorphism
- Leveraging Inheritance in Python
- Polymorphism in Action
- The Diamond Problem and Method Resolution Order
4. Deep Dive: Special Methods
- Magic Methods and Operator Overloading
- Life Cycle of Objects: Constructors and Destructors
- The Rich Comparisons Model and Iterable Objects
5. Python's Approach to Interfaces and Abstract Classes
- The Role of Abstract Base Classes
- Defining and Implementing Interfaces in Python
- Working with Mixins for Code Reusability
6. Exception Handling and OOP
- Catching and Raising Exceptions
- Custom Exception Classes
- Exception Hierarchy and OOP Principles
7. Advanced OOP Concepts and Python
- Metaclasses and the Python Type Model
- Descriptors and Property Management
- Proxy Models and Delegation Patterns
8. Design Patterns and OOP
- Design Patterns in OOP with Python Examples
- Creational Patterns: Implementing Singletons and Factories
- Behavioral Patterns: Strategy, Command, and State
9. Modeling Real-world Problems
- Applying OOP Principles to Real-world Scenarios
- Component-based Design and Service-oriented Architecture
- From Monoliths to Microservices: OOP in System Design
10. Effective OOP with Python Frameworks
- Django's OOP Underpinnings
- Flask and the Minimalist Approach to OOP
- The Async World of Python with OOP
11. Testing and OOP
- Unit Testing of Classes and Objects
- Integration Testing and OOP
- Mocks, Stubs, and Test-driven Development
12. Performance and Optimization in OOP
- Profiling Object-oriented Code
- Memory Management and Optimization
- Concurrency and Parallelism with OOP